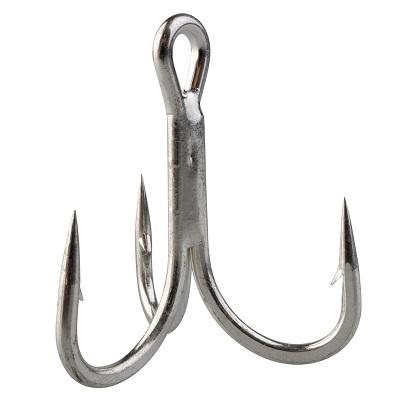
Introducing React Hooks - A blog series
React Hooks are functions that enable functional components to use state and other React features without writing a class. They offer a more elegant and concise way to handle state management, side effects, and other functionalities within functional components, making our code cleaner, more modular, and easier to understand.
Throughout this series, we'll explore each React Hook in-depth, and get to know its capabilities, and demonstrate how it can empower us to build robust and scalable React applications. Here's a sneak peek at the hooks we'll be covering:
-
useState: We'll start our journey with useState, which allows functional components to manage local state, replacing the need for class-based state management.
-
useEffect: Next up is useEffect, a powerful hook for handling side effects in functional components, such as data fetching, subscriptions, or manually changing the DOM.
-
useContext: With useContext, we'll explore how to consume React context within functional components, facilitating global state management and prop drilling avoidance.
-
useRef: useRef opens the door to imperative DOM manipulations and accessing DOM nodes directly within functional components, offering greater flexibility and control.
-
useReducer: Similar to Redux, useReducer provides a way to manage more complex state logic within functional components, offering predictability and scalability.
-
useMemo: We'll dive into useMemo, which optimizes performance by memoizing expensive calculations, preventing unnecessary re-renders. What is memoizing you ask? We'll cover it!
-
useCallback: With useCallback, we'll learn how to memoize callback functions to prevent unnecessary re-renders of child components, improving performance.
-
useImperativeHandle: useImperativeHandle enables functional components to interact with imperative APIs or expose methods to parent components using refs.
-
useLayoutEffect: We'll explore useLayoutEffect, which behaves similarly to useEffect but fires synchronously after all DOM mutations, ensuring accurate measurements and updates.
-
useDebugValue: Finally, we'll uncover useDebugValue, a hook for adding custom labels to custom hooks, aiding in debugging and development.
Additionally, we'll dive into the world of custom hooks, where we'll learn how to create reusable, composable, and shareable pieces of logic that can significantly streamline our React applications.
Whether you're a seasoned React developer or just getting started, this series should equip you with the knowledge and skills to leverage React Hooks effectively in your projects. Stay tuned for my upcoming posts, where we'll dive deep into each hook and unlock their full potential. Happy coding!