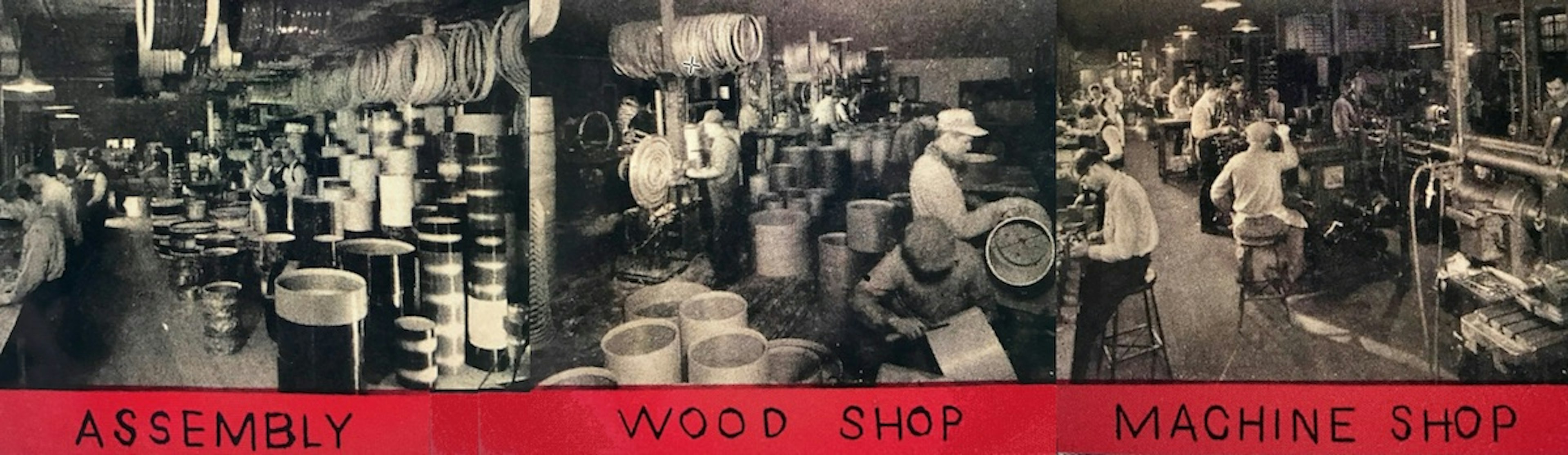
Exploring the Abstract Factory Design Pattern
In the world of software engineering, design patterns are like the building blocks that help developers create scalable, maintainable, and flexible code. One such pattern is the Abstract Factory, which allows for the creation of groups of related objects without specifying their concrete classes. In this post, we'll dive into the Abstract Factory pattern using Ruby, and musical instruments as our business example.
Understanding the Abstract Factory Pattern
The Abstract Factory pattern is a creational design pattern that provides an interface for creating families of related or dependent objects without the need to specify a class for each individual one. This pattern is particularly useful when an application needs to work with multiple families of objects and ensures that the created objects are compatible with each other.
Let's Make Some Music!
Imagine we're building a music store application in Ruby, and we want to model different types of musical instruments, such as brass instruments, drums, and bass guitars. Each instrument category has various types with distinct characteristics.
# Abstract Factory
class InstrumentFactory
def create_instrument(type)
raise NotImplementedError, "#{self.class} does not implement create_instrument method"
end
end
# Concrete Factories
class BrassFactory < InstrumentFactory
def create_instrument(type)
case type
when :trumpet
Trumpet.play
when :trombone
Trombone.play
else
raise ArgumentError, "Invalid instrument type for brass factory: #{type}"
end
end
end
class DrumFactory < InstrumentFactory
def create_instrument(type)
case type
when :drum_kit
DrumKit.play
when :snare_drum
SnareDrum.play
else
raise ArgumentError, "Invalid instrument type for drum factory: #{type}"
end
end
end
class BassFactory < InstrumentFactory
def create_instrument(type)
case type
when :bass_guitar
BassGuitar.play
when :double_bass
DoubleBass.play
else
raise ArgumentError, "Invalid instrument type for bass factory: #{type}"
end
end
end
In the above code, we define an 'abstract' InstrumentFactory
class with a method, create_instrument
, which is responsible for creating instruments. We then create 'concrete' factory classes such as BrassFactory
, DrumFactory
, and BassFactory
, each implementing the create_instrument
method to produce specific types of instruments.
Defining the Musical Instruments
# Abstract Product
class Instrument
def play
raise NotImplementedError, "#{self.class} does not implement play method"
end
end
# Concrete Products
class Trumpet < Instrument
def play
puts "Trumpet: Tooooot!"
end
end
class DrumKit < Instrument
def play
puts "Drum Kit: Boom boom boom!"
end
end
class BassGuitar < Instrument
def play
puts "Bass Guitar: Boom-chicka-boom!"
end
end
Here, we define an abstract Instrument
class with a play
method, which is overridden by concrete instrument classes such as Trumpet
, DrumKit
, and BassGuitar
.
Using the Abstract Factory
Now when we want to implement some type of new logic to be used across all (or some) instruments, we only have to define it once and let our abstract factory class take care of the rest.
def play_music(factory)
instrument = factory.create_instrument
instrument.play
end
# Using the factories
play_music(BrassFactory.new) # Output: Trumpet: Tooooot!
play_music(DrumFactory.new) # Output: Drum Kit: Boom boom boom!
play_music(BassFactory.new) # Output: Bass Guitar: Boom-chicka-boom!
Here, we have a helper method play_music
that takes an InstrumentFactory
as an argument, creates an instrument using the factory, and plays it. We demonstrate the usage of different concrete factories to produce instruments of various types.
So What's The Difference Between The 'Abstract Factory' Pattern and Regular 'Factory' Design Pattern?
The Factory Design Pattern and the Abstract Factory Design Pattern are both creational design patterns, however, they differ in their scope and complexity. The Factory Design Pattern focuses on creating individual objects without specifying their concrete classes, allowing for flexible object creation in a single class. On the other hand, the Abstract Factory Design Pattern extends this concept by providing an interface for creating families of related or dependent objects without specifying their concrete classes, enabling the creation of multiple related objects across different classes. In essence, while the Factory Design Pattern creates single objects, the Abstract Factory Design Pattern creates families of related objects.
Conclusion
The Abstract Factory pattern provides an elegant solution for creating families of related objects in a flexible and decoupled manner. By employing this pattern, we can easily extend our application to support new types of instruments or even entirely different families of objects with minimal changes to existing code. So next time you're faced with a scenario where you need to create lots of objects that are slightly different, yet need similar behaviors, remember the Abstract Factory pattern and get into the app architecture groove! 🎶🎸🥁